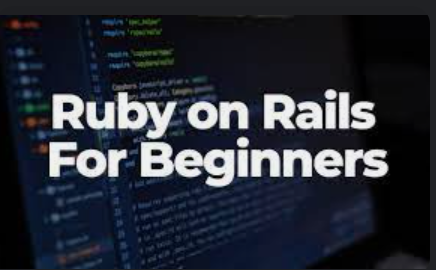
Writing real-life application with automated tests
Writing code & specs in Test-driven development (TDD) flow
Writing model and controller specs in RSpec
Writing service objects (design pattern)
Debugging and fixing bugs in real time
Looking for solutions on your own
Requirements
- Basic knowledge of Ruby
- Basic knowledge of Ruby on Rails framework
Description
After two years of working as a Ruby on Rails developer, I decided to remake my first Ruby on Rails application.
This time I didn’t forget about providing decent test coverage, so you can see how I’m writing specs. You can find a lot of tutorials on how to build different applications quickly, but many are written with any automated tests. Believe me, you don’t write specs for your boss, you write specs for yourself to sleep well while you know that the features you provide are working as expected. If you don’t like specs I’m pretty sure you will change your mind after this course. To be honest I love Ruby on Rails same as RSpec!
I didn’t skip any part when I was looking for solutions using Google or trying to fix bugs I encounter, so you should get a better understanding of how writing Ruby on Rails application looks like in real life.
It’s not always so easy, sometimes looking for the answer takes some time.
Are you ready for the challenge? Ready to become the next RoR & RSpec master? I hope so.
Please check the Course content section to see how many steps this tutorial has to develop the application you can see in Course Preview. These steps are not maybe so well planned as you may see in other tutorials but that’s simply how programming looks like, you can’t plan everything and often you need to improvise and be flexible.
Who this course is for:
- Beginner Ruby on Rails developers curious how to write real-life Ruby on Rails application with decent test coverage
Course content
1 section • 80 lectures • 14h 35m total length
Real-life Ruby on Rails App From Scratch In 14 Hours (RSpec)80 lectures • 14hr 36min
- Introduction04:11
- Create a skeleton of the application05:58
- Create a remote repository on GitHub03:54
- Install RSpec05:10
- Install Haml04:22
- Create Word model17:22
- Change the database from SQLite to PostgreSQL05:03
- Install Simple Form gem02:41
- Create WordsController and basic index view12:47
- Write a spec for WordsController08:31
- Add validation to check the presence of value and language attributes08:35
- Install factory_bot gem08:40
- Refactor spec for WordsController05:42
- Create a view and form to add new words10:47
- Write specs for new and create methods in WordsController24:06
- Install bootstrap05:54
- Set root_path and create show action for single Word with specs09:26
- Rename the project09:07
- Implement basic styling13:01
- Update title and add a link to show view05:29
- Rename column “value” to “content”05:44
- Fix views after renaming the column01:36
- Fix form after renaming the column02:34
- Install language_list gem and add new validation for language field19:19
- Move language string to separate model13:19
- Add relationships between Word and Language models19:10
- Allow editing Word22:21
- Allow deleting Word09:02
- Use before_action in WordsController04:40
- Install Devise gem09:05
- Add links to login, logout, etc05:48
- Only signed-in users can add new words05:31
- Improve spec to test scenarios when a user is signed in or not31:27
- Connect word with user who created it12:52
- Only signed-in users can edit and destroy words13:16
- Prepare seeds11:35
- Implement authorization with pundit gem13:01
- Fix specs after authorization changes08:11
- Write specs for authorization11:41
- Add pagination for words index view13:18
- Install brakeman, bundler-audit gems for security reasons09:43
- Add new relationships to Word model for translations17:11
- Add specs for new relationships between models09:59
- Install nested_form gem and use it for translations15:35
- Write a spec for nested_form stuff in create action22:35
- Display count of translations for each word in the index view10:42
- Do not allow translations to be in the same language as Word15:50
- Implement bootstrap navbar and improve some styling17:44
- Styling improvements to get a decent look36:42
- Hide part of an email address for visitors12:06
- Update rack gem to fix the security vulnerability03:38
- Create Game model08:45
- Fix bug about deleting translations04:34
- Allow user to create new Game12:36
- Write specs for creating new Game by user20:25
- Authorize Game object so only its user can access it06:57
- Add info about good and bad answers to show page04:03
- Create a service object to get random Word21:52
- Query only words with translations when looking for random Word09:57
- Create a service object to check the answer provided by a user10:15
- Write specs for service object checking answers07:16
- Try to use service object to check answers in view32:29
- Update game stats and write new specs for the service object18:28
- Move logic about preparing message to service object15:17
- Write specs for AnswersController17:45
- Make sure that user can access the game06:48
- Improve styling of Game show page13:28
- Make main input autofocus02:23
- Improve the way to notify if the answer was good or bad17:26
- Add a link to continue previous Game06:23
- Add correctness method to Game model22:08
- Fix bug when trying to add new translation in edit view08:12
- Add dependent destroy option04:02
- Show links only if a user can perform the action03:01
- Add confirm prompt before destroying Word02:33
- Show input for first translation at once03:19
- The end01:56
- Improve index query03:01
- Enable Faker gem on production & fix security issues by updating gems08:25
- Fix other security issues by update gems (no sound)06:12