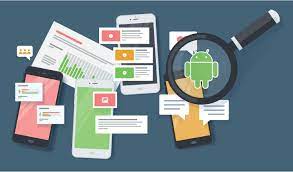
Duration
28 hours (usually 4 days including breaks)
Requirements
None
Overview
Android is an open source platform developed by Google for mobile development.
Applications for the Android platform are developed in Java.
This course overviews Android’s fundamental topics.
This is the fast track program that paces up the original training
Course Outline
Introduction
- What is Android?
- Android SDK
- Android JVM
- The Software Stack
- The Development Tools (ADT)
- User Interface
- Installing Development Tools
- Content Providers
- Services
- Intents
- Activities
- Views
- Configuration File
- Simple Hello World
- Application Artifacts
- Asset Packaging Tool
- Entry Point Activity
- Intent
- Calling Other Activities
- The Activities Stack
- Paused & Stopped Activities
- SQLite Database
- System Management
- Separated Processes
- Component & Integration Architecture
Application Resources
- Introduction
- What are Resources?
- String Resources
- Layout Resources
- Code Samples
- Resource Reference Syntax
- Compiled Resources
- Compiled Animation Files
- Compiled Bitmaps
- Compiled UI View Definitions
- Compiled Arrays
- Compiled Colors
- Compiled Strings
- Compiled Styles
- Compiled Arbitrary Raw XML Files
- Uncompiled Resources
- The .apk File
- Assets
- Assets & Resources Directory Structure
The Intent Concept
- Introduction
- Intent Filter
- Use Intent to Start Activity
- Android Available Intentions
- Code Samples
- Intent Categories
- Late Run-Time Binding
- Use Intent to Start Service
- Broadcast Receivers
- The Intent Object Structure
- The Intent Component Name
- The Intent Action
- The Intent Data
- The Intent Category
- The Intent Extras
- The Intent Flags
- Intents Resolution
- Intent Filter Structure
- The Action Test
- The Category Test
- The Data Test
- Multiple Matches
- Android Predefined Intents
- Samples
User Interface Controls
- Introduction
- GUI Sample in Source Code
- GUI Sample in XML
- GUI Sample in XML & Source Code
- TextView
- TextView Sample
- TextView Style Sample
- EditText
- EditText Sample
- AutoCompleteTextView
- AutoCompleteTextView Sample
- MultiAutoCompleteTextView
- MultiAutoCompleteTextView Sample
- Button
- Button Sample
- ImageButton
- ImageButton Sample
- ToggleButton
- ToggleButton Sample
- CheckBox Control
- CheckBox Control Sample
- RadioButton Control
- RadioButton Control Sample
- ListView
- ListView Sample
- GridView Control
- GridView Control Sample
- Date & Time Controls
- Gallery Controller
- MapView
- WebView
Layout Managers
- Introduction
- LinearLayout
- Layout Weight
- Gravity
- Samples
- TableLayout
- Padding Properties
- RelativeLayout
- AbsoluteLayout
- FrameLayout
- TabsHost
Menus and Dialogs
- Introduction
- Menu Interface
- MenuItem Interface
- SubMenu Interface
- Menu Items Group
- Menu Items Attributes
- Container Menu Items
- System Menu Items
- Secondary Menu Items
- Alternative Menu Items
- Creating Menu
- Sample
- Menu Items Groups
- Menu Items Events Handling
- Overriding Callback Function
- Define Listener
- Using Intents
- Expanded Menu
- Icon Menus
- Sub Menus
- System Menus
- Context Menus
- Samples
- Handling Menu Events
- Creating Menu using XML
- Alert Dialog
- Prompt Dialog
- Samples
Touchscreens
- Introduction
- Motion Events
- Events Sequences
- Events Handling
- Handling Method
- Samples
Style Definition
- Introduction
- Style Definition
- Samples
- Style Definitions Inheritance
- Style Properties
- Theme Definition
- Predefined Themes
- Predefined Themes
- Inherit Predefined Theme
- App Widgets Development
- App Widgets Overview
- Practical Samples
- Basic App Widget Structure
- Configuration Activity
- Design Guidelines
Location Based Services
- Introduction
- The Map Key
- The MD-5 Signature
- Google Maps Key
- Required Permissions
- Code Sample
- The Map Controller
- Code Samples
- Maps Overlays
- Code Samples
- The Geocoder Class
- The Address Class
- The LocationManager Class
- The LocationListener Interface
- The Debug Monitor Service (DMS)
Web Services
- Introduction
- The HttpClient Class
- The HttpGet Class
- The HttpPost Class
- The HttpResponse Class
- Code Samples
- HTTP Get Request
- HTTP Post Request
- Code Samples
- Timeout Exceptions
- Threading Issues
Android Services
- Introduction
- Creating Services
- The Service Class
- Background Tasks
- Inter Process Communication
- Separated Implementation
- Local ServiceRemote Services
- AIDL Compiler
- Creating Remote ServiceUsing Remote Service
- Samples
Media Framework
- Introduction
- Playing Audio
- Playing Video
- Simple MP3 Player Demo
- Simple Video Player Demo
Android Security Model
- Introduction
- Deployment
- The keytool Utility
- The jarsigner Utility
- Deployment using Eclipse
- Separated Processes
- Declarative Permission Model
Graphics Animation
- Introduction
- Frame by Frame Animation
- Code Sample
- Tween Animation
- Code Samples
Basic Graphics
- Introduction
- The Drawable Abstract Class
- Code Sample
- The ShapeDrable Abstract Class
- Code Sample
OpenGL Graphics
- Introduction
- The glVertexPointer Method
- The glDrawElements Method
- Code Sample
Customized Views
- Introduction
- Customized Progress Bar
- Model View Controller
Android Threads
- Introduction
- The UI Thread
- The Single Thread Rule
- The runOnUiThread Method
- The post Method
- The postDelayed Method
- The Handler Class
- The AsyncTask Utility Class
- Sending Messages to Handler
- Background Threads Caveats
Application Life Cycle
- Introduction
- Activity Life Cycle Methods
- The onStart() and onResume() Methods
- The onPause() and onStope() Methods
- Return Back to Previous Activity
- The onStop() and onDestroy() Methods
- The onCreate() Method
- The onPause() Method
SQLite Database
- Introduction
- SQLite Implementation
- The SQLiteOpenHelper Class
- The onCreate() Method
- The onUpgrade() Method
- The onOpen() Method
- The getWriteableDatabase() Method
- The getReadableDatabase() Method
- The SQLiteDatabase Class
- The execSQL() Method
- The insert() Method
- The delete() Method
- The rawQuery() Method()
- Code Samples
- The query() Method
- Code Samples
Content Providers
- Introduction
- Android Built-In Content Providers
- SQLite Database
- Content Providers Architecture
- Content Providers Registration
- Content Providers REST Access
- Content Providers URL Structure
- Content Providers Mime Types
- Using Content Provider
- Retrieving Records,Adding Records
- The Cursor Object,The ContentValues() Object
- Content Provider Demo
- Developing Content Providers
- Code Samples
- The SimpleCursorAdapter Class
- The onCreate() Method
- The query() Method
- The insert() Method
- The update() Method
- The delete() Method
- The getType() Method
- Code Samples
- Changes Notification
Shared Preferences
- Introduction
- The SharedPreferences Interface
- The SharedPreferences.Editor Class
- Code Samples
File Management
- Introduction
- Creating Files
- Accessing Simple Files
- Accessing Raw Resources
- XML Files Resources
- SD Card External Storage
Dalvik VM
- Introduction
- Comparing with JVM
- The dex File Format
- The dx Utility
- Garbage Collector
- Controlling the Dalvik VM
Background Applications
- Introduction
- Services
- Background Threads
- Making Toasts
- Notifications
- Other System Services
- Background Activity Sample
Activity Data
- Introduction
- The Intent Class
- Start Activity Methods
- Passing Data between Activities
- Coherent User Experience
- Code Sample
SMS Messages
- Introduction
- The SMSManager Class
- The SEND_SMS Permission
- Sending SMS
- Receiving SMS
Telephony
- Introduction
- Initiate Phone Calls
- The android.telephony Package
- The CallLocation Class
- The PhoneStateListener Class
- The ServiceState Class
- The TelephonyManager Class
- Limitations,Android VoIP
Web View
- Introduction
- The WebView Class
- The android.webkit Package
- The INTERNET Permission
- The loadUrl() Method
- JavaScript Support
- The loadData() Method
- The WebView Methods
- The WebViewClient Class
- The WebChromeClient Class
Java Language
- Introduction
- The Limits
- Third Party Java Libraries
Debugging
- Introduction
- Eclipse Java Editor
- Eclipse Java Debugger
- Logcat
- Android Debug Bridge
- Dalvik Debug Monitor Service
- Traceview
- Instrumentation Framework
Adapters
- Introduction
- Classes Hierarchy
- The Adapter Purpose
- The SimpleCursorAdapter Class
- The ArrayAdapter Class
Live Wallpapers
- Introduction
- Develop Live Wallpaper
- User Interaction
- The user-sdk Element
- The uses-feature Element
- PerformanceCode Samples
BroadcastReceiver
- Introduction
- Registering a Receiver
- Receiver Life Cycle
- Code Samples
Bluetooth
- Introduction
- The Capabilities
- The BluetoothAdapter Class
- The BluetoothDevice Class
- The BluetootnSocket Interface
- The BluetoothServerSocket Class
- The Bluetooth Class
- Bluetooth Permission
- Setting Up Bluetooth
- Finding Devices
- Querying Paired Devices
- Devices Discovery
- Enabling Discoverability
- Devices Connection
- Code Samples
Text To Speech
- Introduction
- Pico Engine
- Demo
- Single TTS Engine
- Code Samples
Android Search
- Introduction
- Suggestions Providers
- The onSearchRequest() Method
- Suggestion Provider
Camera
- Introduction
- Auto Focus
- Picture Parameters
- Scene Modes
Accelerometer
- Introduction
- The SensorManager Class
- The SensorListenr Interface
Compass
- Introduction
- The SensorManager Class
- The SensorListenr Interface
Vibration
- Introduction
- The Vibrator Class
- Vibration Sequences
WiFi Connectivity
- Introduction
- The WifiManager Class
- User Permissions
- Searching for Hot Spots
- Connecting Hot Spots
Networking
- Introduction
- Java Networking Classes
- Android Networking Classes
- Apache Networking Classes
Input Method Framework
- Introduction
- The android:inputType Attribute
- Customized IMF
Device Rotation
- Introduction
- Two XML Layout Documents
- Code Samples
Localization
- Introduction
- Default Resources
- Current Locale
- Testing
- Custom Locale
- Code Samples
Memory Management
- The Application Context
- Screen Orientation Changes
- Static Inner Classes
- Weak References
- Garbage Collector
Speech Input
- Introduction
- The RecognizerIntent Class
- Start Speech Recognition
- Google Server Side
- The Language Model
- Free Form Language Model
- Web Search Language Model
Development Tools
- Introduction
- The aapt Tool
- The adb Tool
- The android Tool
- The ddms Tool
- The dx Tool
- The draw9patch Tool
- The emulator Tool
Instant Messaging
- Introduction
- Jabber Protocol
- GTalk Interaction