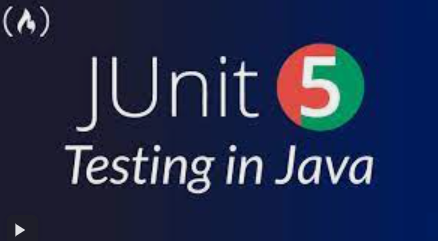
Understand JUnit architecture
Understand and create tests using assertions with hands on coding
Create different types of tests – repeated, conditional, nested etc…
Create TestSuites
Generate JUnit reports
Requirements
- Basic Java knowledge
Description
This course is for Java developers who are trying to learn JUnit5 to test their code.
JUnit is probably the most widely used unit testing framework for Java developers. It is the next iteration of JUnit after Junit4 and has a number of new features added.
Knowing how to test your code automatically and enabling your code to be seamlessly tested as part of a CICD pipeline is a “must have” for any developer.
With this course you learn:
JUnit 5 architecture – You learn the basic architecture of JUnit5 and how it builds on top of the JUnit testing platform.
Lifecycle annotations – @Test, @BeforeEach, @AfterEach, @AfterAll @BeforeAll
Testing using assertions – You learn to test for equality, null, exceptions etc…
Various testing scenarios – Write test cases using @RepeatedTest, @ParameterizedTest, @ConditionalTest
Grouping tests – group various tests using @Nested and @Suite annotations.
Reporting test results using maven. With just a few additions to your maven configuration, you will be able to publish these results to a web-page and also share it with your team.
All of the coding will be done on Eclipse. However, if you are familiar with IntelliJ, that should be fine too.
The course will be completely hands on, except when it is required to explain concepts.
Who this course is for:
- Java developers and anyone interested in building robust Java applications
Course content
8 sections • 21 lectures • 49m total lengthExpand all sections
Course Introduction1 lecture • 2min
- Introduction01:34
JUnit5 Architecture and Environment Setup2 lectures • 4min
- JUnit Architecture01:56
- Environment Setup01:56
Your first JUnit test3 lectures • 7min
- Section Introduction00:36
- Creating test cases with a custom folder structure02:43
- Creating test cases using Maven03:54
JUnit lifecycle and assertions7 lectures • 14min
- JUnit Lifecycle annotations01:07
- Assertions Introduction00:56
- Assertions – 102:32
- Assertions – 201:57
- Assertions – 302:35
- Before and After annotations04:11
- Naming Tests01:08
Types of JUnit testing4 lectures • 15min
- Enable and Disable Tests02:42
- Ordering the sequence of test cases01:44
- Repeated tests03:05
- Parameterized tests07:19
Grouping tests2 lectures • 5min
- Grouping tests using @Nested01:20
- Grouping tests using @Suite03:41
Reporting test results1 lecture • 2min
- Reporting using maven site02:22
Conclusion1 lecture • 1min
- Thank you!00:21