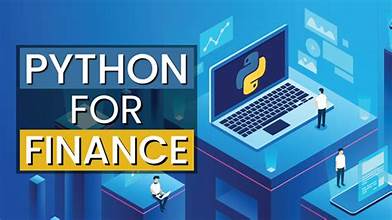
Introduction
Setting up the Development Environment
- Programming locally vs online: Anaconda and Jupyter
Python Programming Fundamentals
- Control structures, data types, functions, data structures and operators
Extending Python’s Capabilities
- Modules and Packages
Your first Python Application
- Estimating beginning and ending dates and times
Accessing External Data with Python
- Importing and exporting, reading and writing CSV data
- Accessing data in an SQL database
Organizing Data Using Arrays and Vectors in Python
- NumPy and vectorized functions
Visualizing Data with Python
- Matplotlib for 2D and 3D plotting, pyplot, and SciPy
Analyzing Data with Python
- Data analysis with scipy.stats and pandas
- Importing and exporting financial data (Excel, website data, etc.)
Simulating Asset Price Trajectories
- Monte Carlo simulation
Asset Allocation and Portfolio Optimization
- Performing capital allocation, asset allocation, and risk assessment
Risk Analysis and Investment Performance
- Defining and solving portfolio optimization problems
Fixed-Income Analysis and Option Pricing
- Performing fixed-income analysis and option pricing
Financial Time Series Analysis
- Analyzing time series data in financial markets
Taking Your Python Application into Production
- Integrating your application with Excel and other web applications
Application Performance
- Optimizing your application
- Parallel Computing and Multiprocessing
Troubleshooting
Closing Remarks