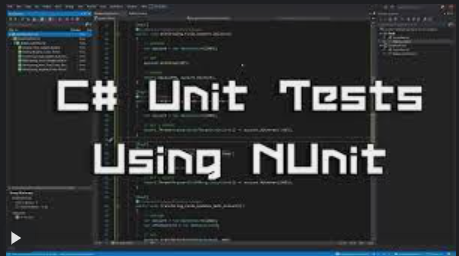
Write simple unit tests in C# using the NUnit framework
Requirements
- Basic Skills in C#
Description
This course is all about writing unit tests using C# programming language and NUnit as a unit testing framework. Today unit testing is an absolutely required skill from any professional developer. Companies expect from developers to know how to write unit tests.
Learning unit testing puts a powerful and very useful tool at your fingertips. Being familiar with unit testing you can write reliable and maintainable applications. It is very hard to lead a project which is not covered by unit tests.
Content and Overview
This course is primarily aimed at beginner developers.
We start with basics of unit testing. What is a unit test? What unit testing frameworks exist? How to run and debug unit tests. After getting acquainted with the basics, we will get to the NUnit framework. Here you’ll learn how to install the framework, set the runner. Then you’ll learn the basics of assertions and arrange-act-assert triplet. Other key features of NUnit are also covered:
- Running tests from the console
- Setup and teardown unit tests
- Parameterized tests
- Grouping and ignoring
Who this course is for:
- Anyone who wants to learn the basic of unit testing
Course content
2 sections • 34 lectures • 1h 52m total lengthExpand all sections
Getting Started16 lectures • 47min
- About the Course00:27
- Download Source Code and Slides00:04
- Join .NET Community of Students00:02
- BONUS00:35
- Outline00:58
- What is a Unit Test?03:11
- Unit-Testing Frameworks03:25
- First Unit Test08:49
- Naming Conventions04:34
- Running and Debugging Tests in Visual Studio05:02
- Benefits of Unit Testing03:35
- Who should write unit tests and When?02:51
- Programmer’s Oath03:29
- Exercise: Degree Converter01:29
- Solution: Degree Converter06:54
- Conclusion01:15
NUnit Framework18 lectures • 1hr 6min
- Outline01:06
- Assert. Introduction05:29
- Assert. Demo10:27
- Arrange-Act-Assert (AAA)01:01
- Running a Test from the Console01:51
- SetUp and TearDown05:09
- SetUp and TearDown on Higher Levels02:13
- Parameterized Tests03:40
- Grouping and Ignoring02:14
- Code Coverage01:42
- Exercise: FizzBuzz01:07
- Solution: FizzBuzz05:05
- Exercise: Roman Numerals02:27
- Solution: Roman Numerals09:27
- Exercise: Stack00:59
- Solution: Stack09:02
- Conclusion01:24
- BONUS-Video01:18